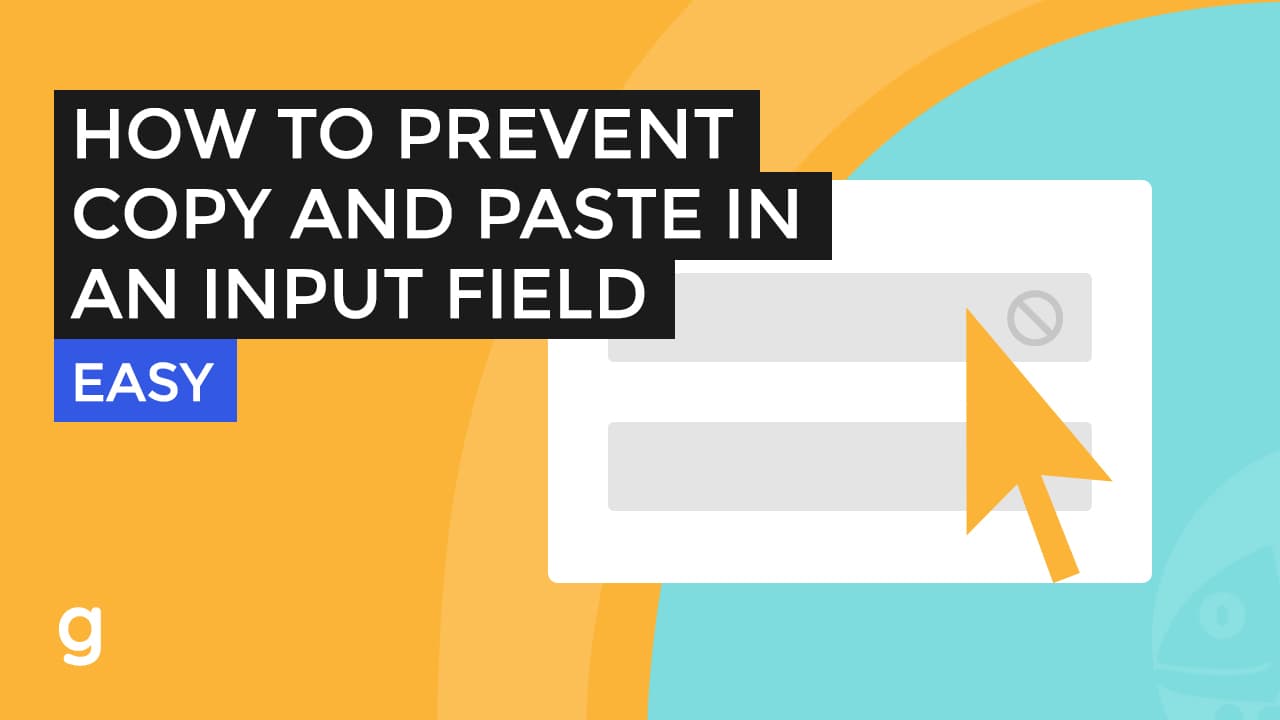
On the following html code, you will see there are 2 input fields. One with a type of email and the other one is password.
<input type="email" id="email" pattern=".+@globex\.com" required> <br><br> <input type="password" id="pass" name="password" minlength="6" required >
Disabling Copy and Paste using HTML Attributes
Disable it by adding an onpaste attribute with a value of return false.
“onpaste” is an HTML event attribute that can be added to an element. It triggers a script to run when the element is pasted into, which happens when a user pastes content into it. If the script that runs is “return false;”, then the paste action is blocked, and no content is pasted into the element.
<input type="email" id="email" onpaste="return false;" pattern=".+@globex\.com" required> <br><br> <input type="password" id="pass" onpaste="return false;" name="password" minlength="6" required >
Now let’s disable any drag-and-drop content on the fields.
Add an ondrop attribute with a value of return false.
The “ondrop” attribute contains a JavaScript statement, “return false;”, that is executed when a drop event occurs on an HTML element. This statement prevents the default action of the drop event from taking place.
<input type="email" id="email" onpaste="return false;" ondrop="return false;" pattern=".+@globex\.com" required> <br><br> <input type="password" id="pass" onpaste="return false;" ondrop="return false;" name="password" minlength="6" required >
Disabling Copy and Paste using JavaScript
Let’s declare a constant variable called “inputField” and assigns it the value of the element with an ID of “email” from the HTML document.
Next, let’s add an event listener to the “inputField” element for the “paste” event.
The “paste” event occurs when the user pastes content into the element. The function specified by the event listener will be called whenever this event occurs.
The function calls the “preventDefault” method on the event object, which cancels the default action of the “paste” event. This means that if the user tries to paste content into the element, the default action of inserting the pasted content into the element will be prevented.
This code attaches a function to an element with the ID “email” that will be triggered when the user attempts to paste the content into the element. The function cancels the default behavior of inserting the pasted content into the element.
const inputField = document.getElementById('email'); inputField.addEventListener('paste', e => e.preventDefault());